Dive into the world of Python web development with our comprehensive Flask tutorial! Whether you’re a beginner or an experienced developer, this step-by-step guide covers everything you need to know. From understanding the fundamentals of Flask to setting up and installing the framework, creating and running your first Flask app, and even building a robust REST API тАУ we’ve got you covered. Follow along with practical examples and hands-on exercises to enhance your skills and become a Flask master. Get ready to build a REST API in minutes with this ЁЯФе Python web development tutorial!
Table of Contents
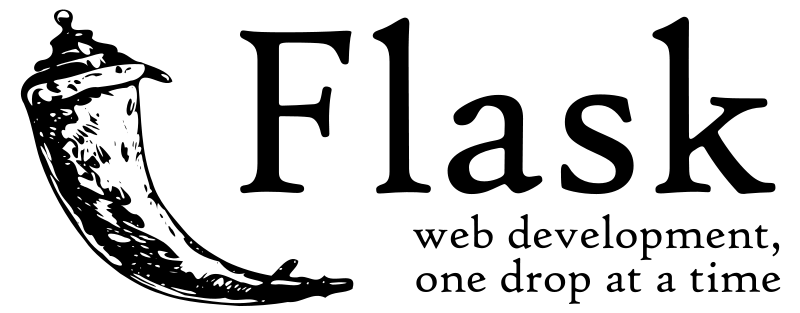
Flask Introduction:
Flask is a lightweight web application framework for Python. It’s designed to be simple and easy to use, making it a popular choice for building small to medium-sized web applications and APIs. Flask provides the essentials for web development without imposing too many constraints on the developer.
Key features of Flask include:
- Routing: Flask allows you to define routes, which determine how the application responds to different URL patterns.
- Templates: Flask uses Jinja2 templates to render dynamic content and generate HTML pages.
- Web Forms: Flask includes support for handling web forms, making it easier to work with user input.
- HTTP Request/Response Handling: Flask simplifies handling HTTP requests and responses, including methods like GET and POST.
- Extensions: Flask has a modular design and supports extensions, allowing you to add additional features as needed.
- Development Server: Flask comes with a built-in development server, making it easy to test your applications during development.
Sample Code
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def hello():
return ‘Hello, World!’
if __name__ == ‘__main__’:
app.run(debug=True)
In this example, a basic Flask application is created with a single route (“/”) that responds with “Hello, World!” when accessed. To run this application, you would typically save it in a file (e.g., app.py
) and execute it. The development server would then start, and you could access the application in your web browser.
Flask is often used in conjunction with other libraries and tools, such as SQLAlchemy for database integration, WTForms for form handling, and Flask-RESTful for building RESTful APIs. Its simplicity and flexibility make it a popular choice for many developers working on web projects with Python.
How to install flask?
To install Flask, you can use the Python package manager, pip
. Here are the steps to install Flask:
- Open a Terminal/Command Prompt:
- On Windows, you can open the Command Prompt or PowerShell.
- On macOS or Linux, you can use the Terminal.
- Install Flask: Run the following command to install Flask using
pip
:pip install Flask
- If you are using Python 3, you might need to use
pip3
instead:
- If you are using Python 3, you might need to use
pip3 install Flask
- Verify the Installation: After the installation is complete, you can verify that Flask is installed by checking its version:
flask --version
- This command should display the Flask version installed on your system.
That’s it! Flask is now installed on your machine. You can start building your web applications using Flask by creating Python scripts and defining routes and views as needed. If you encounter any issues during installation, make sure that you have Python and pip
installed correctly, and your system’s PATH variable is set up appropriately.
How to render templates or html file?
In Flask, you can use the render_template
function to render HTML templates. Here’s a simple example to demonstrate how to render an HTML file using Flask:
Create a Flask App: Create a new Python script, for example, app.py
, and include the following code:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
# Render the 'index.html' template
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
Create a Templates Folder: Create a folder named templates
in the same directory as your app.py
file. Flask expects to find templates in a folder named templates
by default.
Create an HTML Template: Inside the templates
folder, create an HTML file named index.html
with some content. For example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Flask Template Example</title>
</head>
<body>
<h1>Hello, Flask!</h1>
<p>This is a simple Flask template example.</p>
</body>
</html>
Run the Flask App: Open a terminal or command prompt, navigate to the directory containing app.py
, and run the application:
python app.py
Visit http://127.0.0.1:5000/
in your web browser, and you should see the content of the index.html
template rendered by your Flask application.
The render_template
function looks for templates in the templates
folder by default, but you can specify a different folder by providing the template_folder
parameter when creating the Flask app instance if needed. This example is just a basic introduction; you can include variables and logic in your templates, making them dynamic and powerful.
How to create rest API?
Create a new Python script, for example, app.py
, and include the following code:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/hello', methods=['GET'])
def hello():
return jsonify({'message': 'Hello, this is a REST API!'})
if __name__ == '__main__':
app.run(debug=True)
In this example, we’ve defined a single route /api/hello
that responds to HTTP GET requests with a JSON message.
Visit http://127.0.0.1:5000/api/hello
in your web browser or use a tool like curl
or Postman to send a GET request, and you should receive a JSON response.
{"message": "Hello, this is a REST API!"}
Expand the API: You can expand your API by adding more routes, handling different HTTP methods (GET, POST, PUT, DELETE, etc.), and integrating with databases or external services.
Here’s a simple example with a route that accepts parameters:
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route('/api/greet', methods=['GET'])
def greet():
name = request.args.get('name', 'Guest')
return jsonify({'message': f'Hello, {name}!'})
if __name__ == '__main__':
app.run(debug=True)
Now, you can visit http://127.0.0.1:5000/api/greet?name=Mukund
to get a personalized greeting.
Remember, this is a basic example, and in a real-world scenario, you would likely want to handle request validation, error handling, and potentially use Flask extensions like Flask-RESTful for more structured API development.
Video Example:
Source Code:
https://github.com/mukund720/flaskDemo